Authenticating with Rollout
Partners authenticate with Rollout by means of a privately signed JWT, using the HS512 algorithm.
In order to generate the signed JWT, you'll need your Client Id
and Client Secret
which can be found under Configuration in the Rollout dashboard.
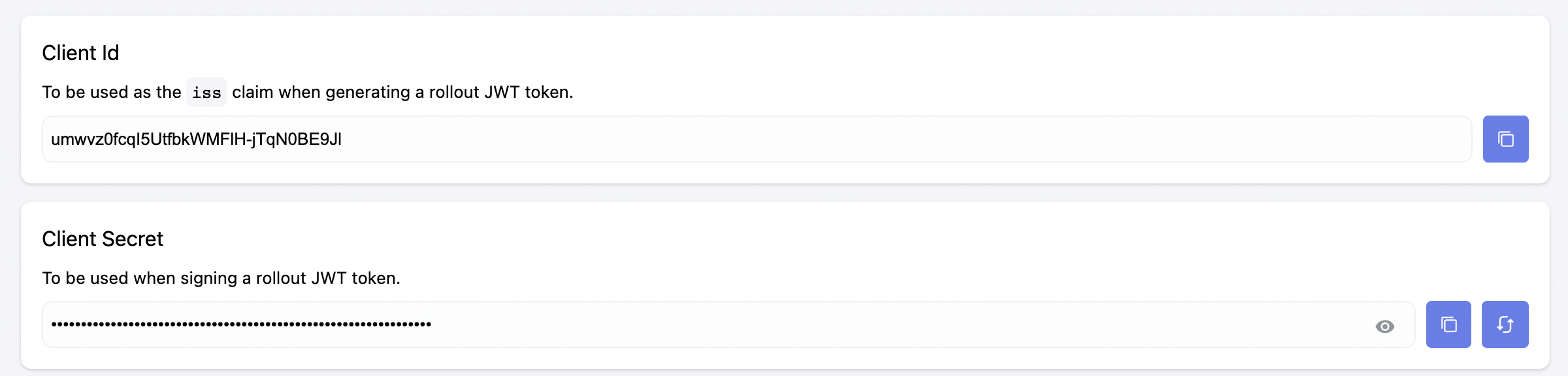
You'll use the Client Secret in order to sign the JWT, while your Client Id
should be the iss
claim of the JWT (see the table below for all of the Rollout JWT's claims).
Make sure to always generate Rollout JWTs on your server in order to keep your Client Secret secure
JWT claims#
Claim | Required | Type | Description |
---|---|---|---|
iss | ✅ | string | Issuer: The Client Id you copied from the Rollout Dashboard |
sub | ✅ | string | Subject: A unique Id for a user or account (i.e. the user viewing the Rollout UI component or the user releavnt to a given API request). Should be consistent accross components and requests pertaining to a given user |
iat | ✅ | integer | Issued At: UNIX timestamp denoting when the token was issued |
exp | ✅ | integer | Expires: UNIX timestamp denoting when the token expires |
rollouthq.com | object | See below |
Rollout-specific claims#
The non-standard, Rollout-specific claims can be passed in under the rollouthq.com
key.
Claim | Required | Type | Description |
---|---|---|---|
dev | boolean | Developer Mode: If present and true , indicates that unpublished Triggers and Actions will be displayed in UI Components | |
authData | object | In case you are using apiKey authorization, this is where you can pass in the auth data that will be available for action executions |
Token Generation Javascript Code Example#
const TOKEN_TTL_SECS = 3600;function generateRolloutConnectToken(userId) {const nowSecs = Math.round(Date.now() / 1000);return jsonwebtoken.sign({iss: process.env.ROLLOUT_CLIENT_ID, // Provided in the Rollout dashboardsub: userId, // Persistent identifier for the consuming user. Must be a stringexp: nowSecs + TOKEN_TTL_SECS, // Token expiration},process.env.ROLLOUT_CLIENT_SECRET, // Provided in the Rollout dashboard{ algorithm: "HS512" });}