Prefilling Automation Form data
You may want to pre-fill data, for example trigger data, by adding default values.
The prefilled
prop can be passed to the components rendering Automation Form in order to specify default values.
For example, this will prefill the automation name:
const prefilled = {name: "prefilled name",};<AutomationCreator prefilled={prefilled} />;
The next example will make user only configure the action. The trigger is preselected to be that of your app. You can find your app key on the Configuration page.
const prefilled = {trigger: {appKey: "admin",triggerKey: "taskCompleted",inputParams: {// Optional input fields for the trigger to prefillinputField: "testValue",},},};<AutomationCreator prefilled={prefilled} />;
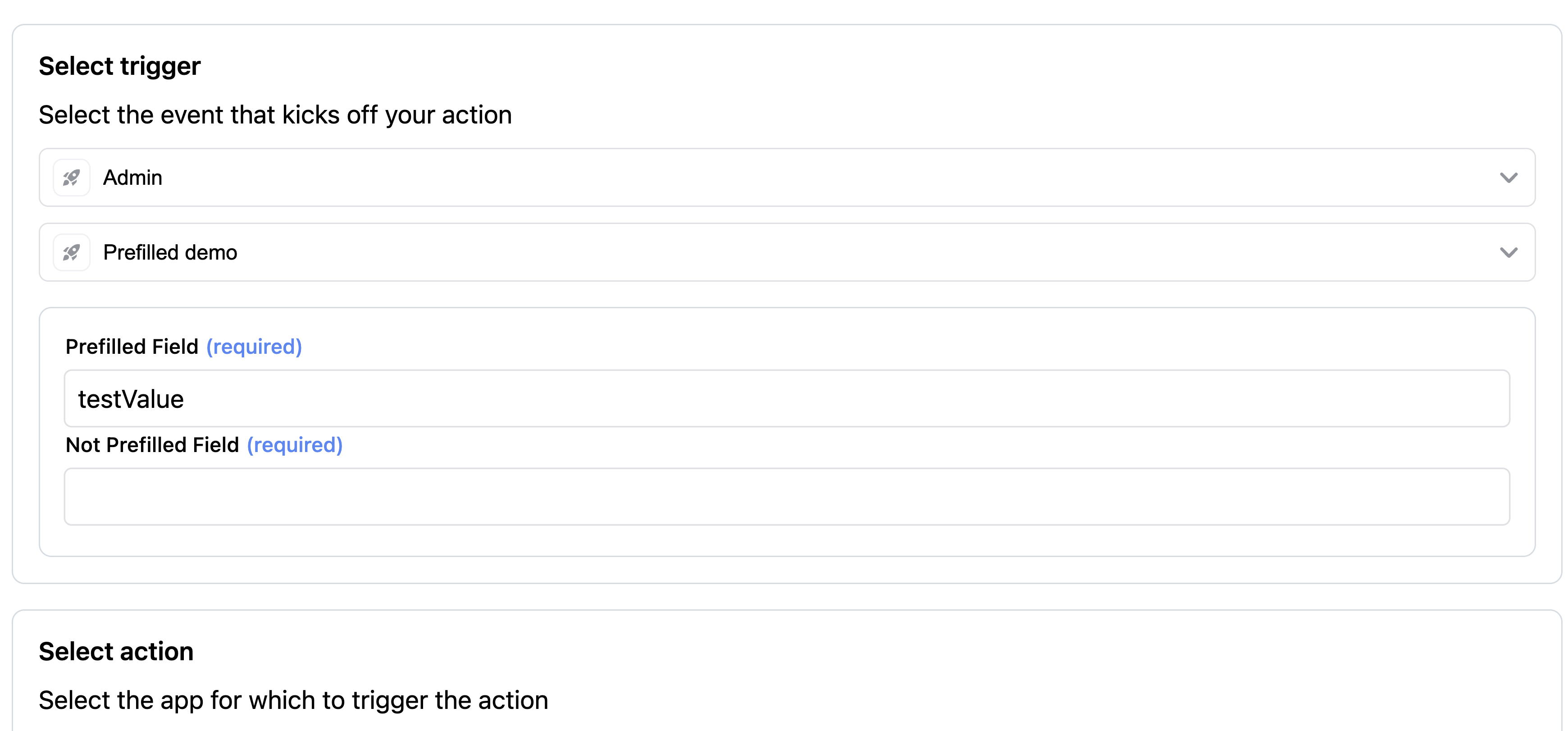
You could also configure the automations to have a third party trigger:
const prefilled = {trigger: {appKey: "asana",triggerKey: "taskAdded",inputParams: {// Optional input fields for the trigger to prefillworkspace: "some_id",},},};<AutomationCreator prefilled={prefilled} />;
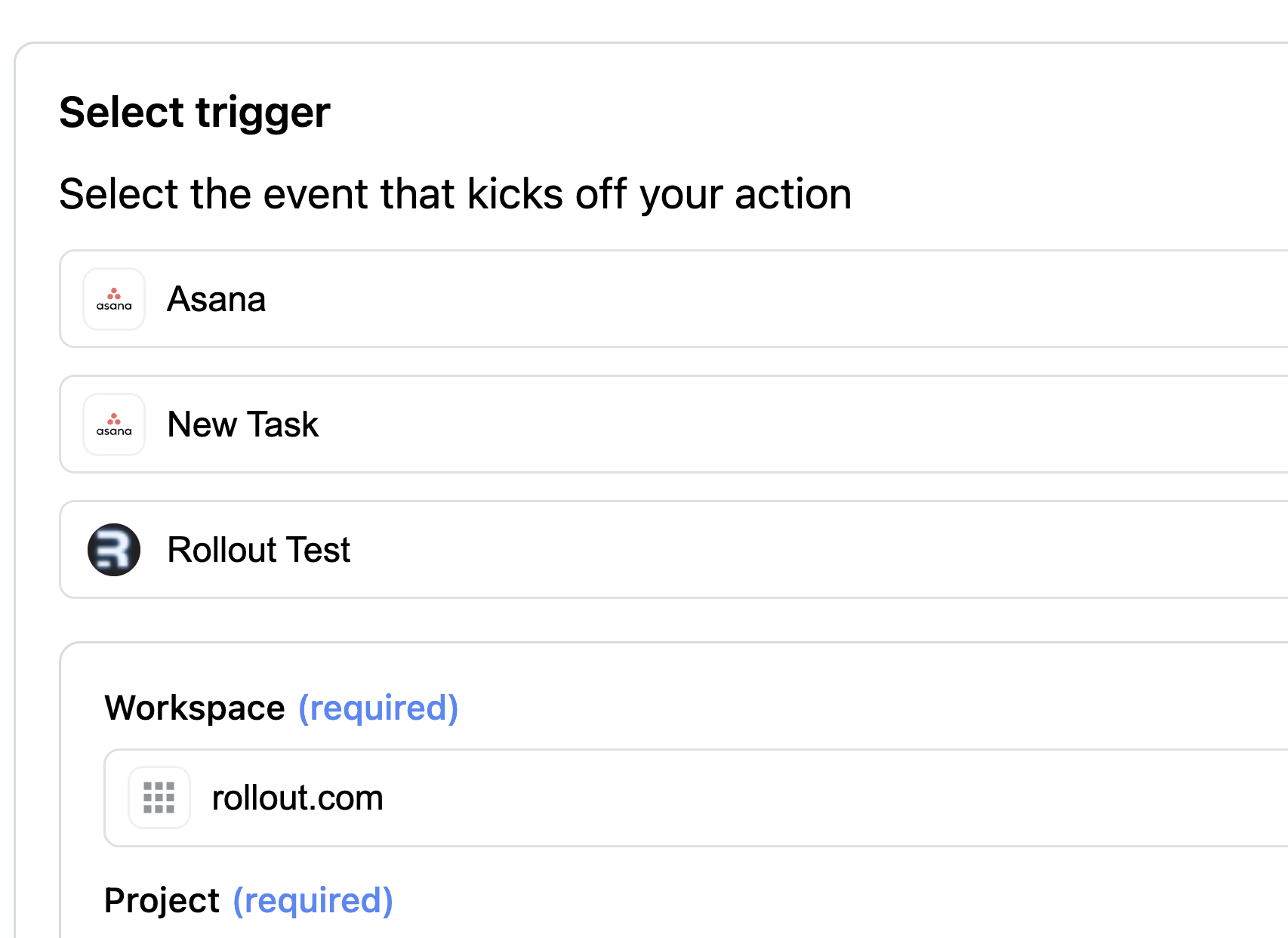
Prefilling Actions#
In the example below, the AutomationCreator
component is configured so that the Asana Create Task action is preselected. You can find a list of all third party actions here.
const prefilled = {trigger: {appKey: "admin",},action: {appKey: "asana",actionKey: "createTask",},};<AutomationCreator prefilled={prefilled} />;
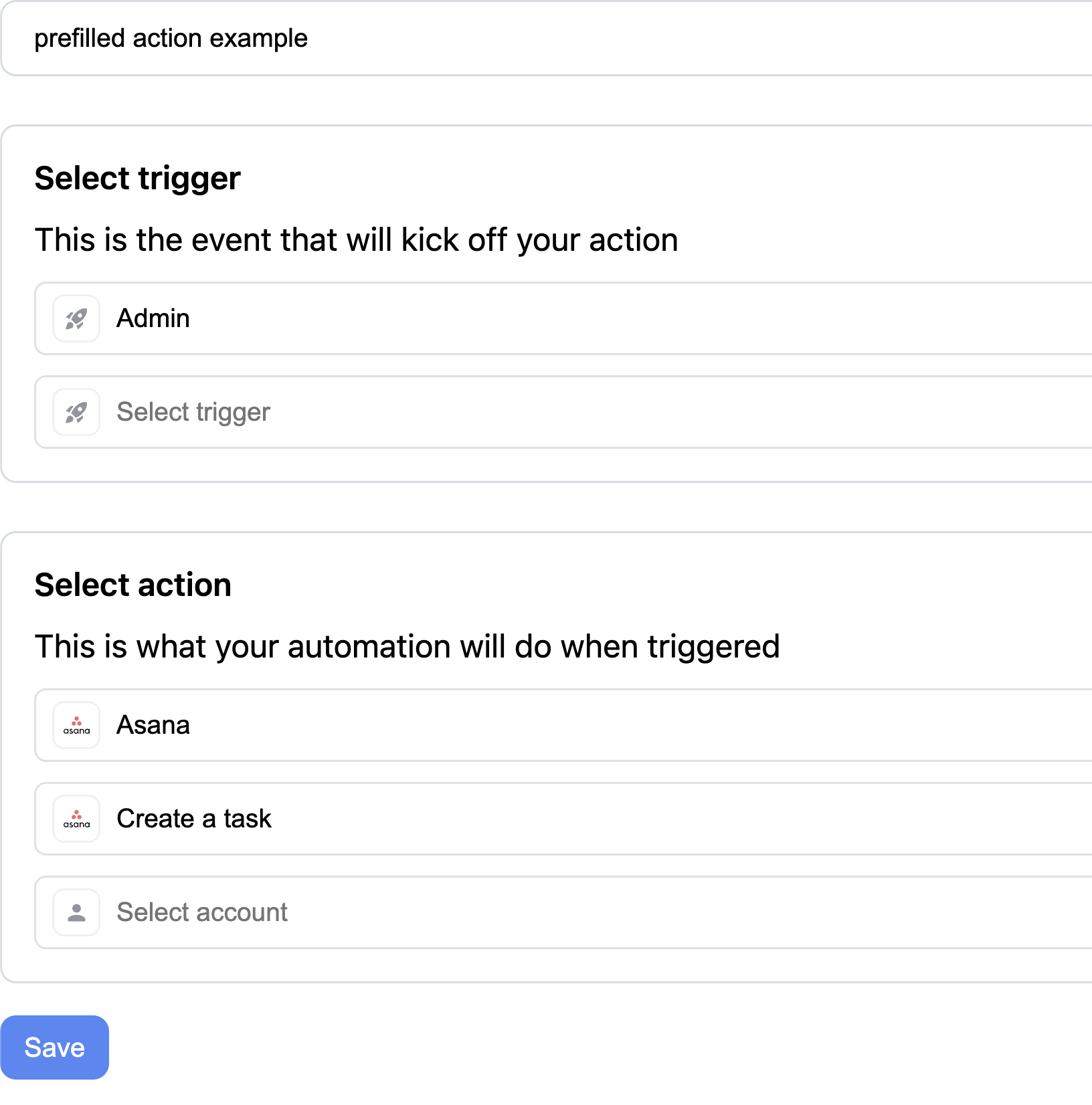
Dynamically Prefilling Actions#
In case the actionKey
is not known ahead (prefilled), or you need to compute the default input params dynamically, you can use the getDefaultActionInputParams
prop which accepts callback function.
The callback is called with the current Automation Form data, so you can access things like currently selected action, or trigger.
The following example does:
- prefill the trigger to
taskCreated
in my app - prefill Asana as the action app
- hide the action app input
- prefill Asana action input params depending on the selected action
import { AutomationCreator } from "@rollouthq/connect-react";const prefilledAsanaInputParams = {createTask: {// Prefill the `name` param to reference the `name` prop from triggername: "{{name}}",},updateTask: {name: "{{name}}",},deleteTask: {// Prefill the `taskId` param to reference the `id` prop from triggertaskId: "{{id}}",},};<AutomationCreatorprefilled={{trigger: {appKey: "myApp",triggerKey: "taskCreated",},action: {appKey: "asana",},}}renderFields={{trigger: false,action: {appKey: false,},}}getDefaultActionInputParams={(form) =>prefilledAsanaInputParams[form.action.actionKey]}/>;
Dynamically Prefilling Triggers#
Similarly to getDefaultActionInputParams
, the getDefaultTriggerInputParams
prop can be used to dynamically prefill trigger input params:
import { AutomationCreator } from "@rollouthq/connect-react";const prefilledTriggerInputParams = {taskAdded: {workspace: "my-workspace",},taskChanged: {workspace: "my-workspace",},};<AutomationCreatorprefilled={{trigger: {appKey: "myApp",},}}renderFields={{trigger: {appKey: false,},}}getDefaultActionInputParams={(form) =>prefilledTriggerInputParams[form.action.actionKey]}/>;